Authorization Protocol
How to implement the OAuth2
Before reading or writing data with APIs, an application needs to authenticate.
Our APIs implements the OAuth 2 authorization protocol and respects its RFC.
The goal of OAuth 2 is to provide a secure and standardized way Klaxoon users to grant selectively your application access to their Klaxoon resources without giving away their login credentials.
The user is presented with a consent screen that explains what your application is requesting access to. Once the user grants authorization, your application is issued an access token, which it can use to access the user's resources.
Overall, the goal of OAuth 2 is to provide a secure and standardized way for third-party applications to access a user's resources while preserving the user's privacy and control over their data.
OAuth 2 Authorization Code Flow
The authorization code flow is the most common flow for a secure authorization. It allows the end user to provide an explicit consent to provide her/his data to a third party application.
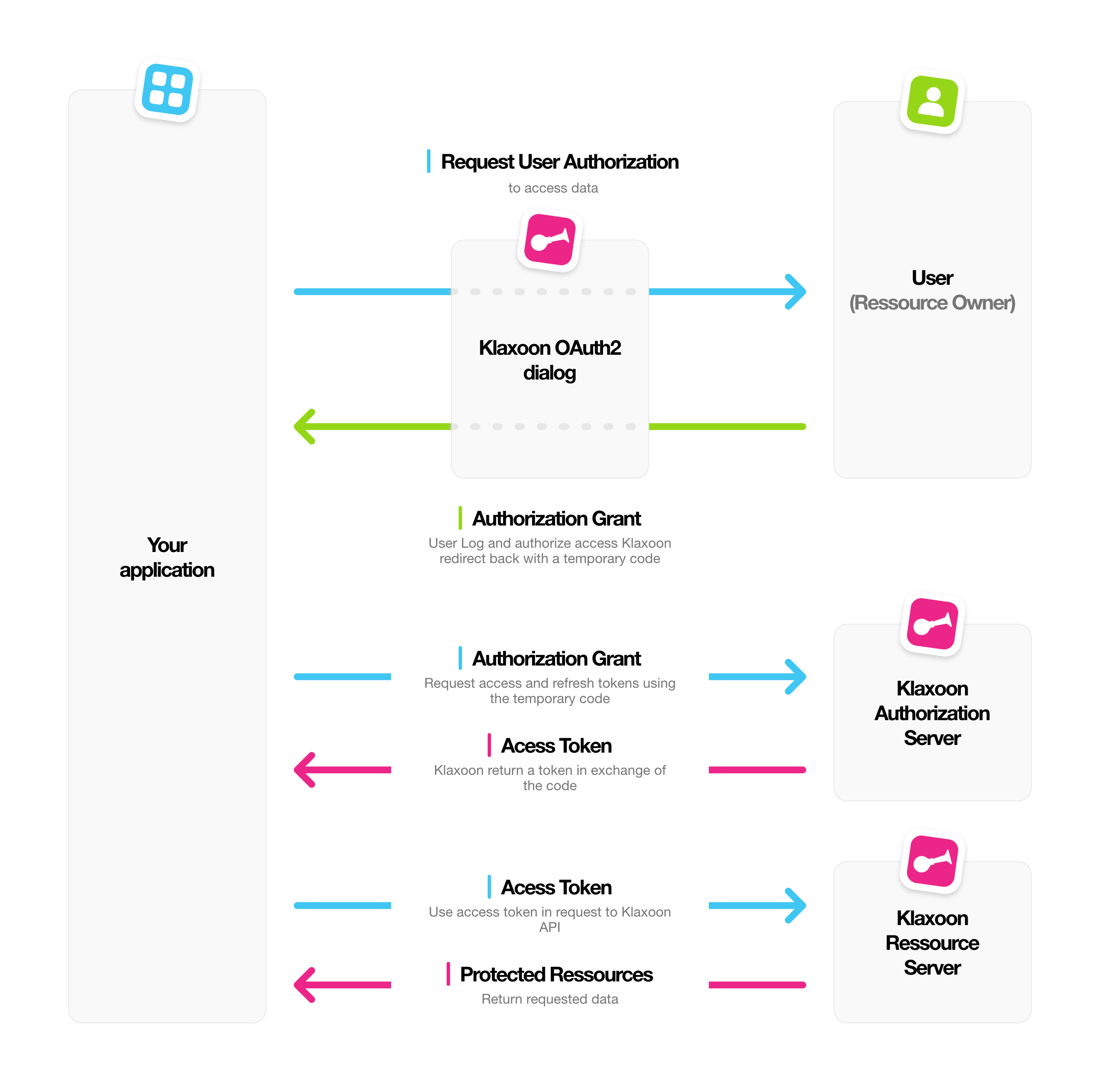
OAuth 2 Authorization Code Flow
The implementation of this authentication flow can be done by following the steps below.
1. Request User Authorization
Your application calls the browser and redirects the user to an OAuth2 dialog.
https://app.klaxoon.com/authorize?
response_type=code
&client_id=[YOUR_CLIENT_ID]
&redirect_uri=[YOUR_REDIRECT_URI]
&scope=[SCOPE_COMMA_SEPARATED]
&state=[SOME_ARBITRARY_BUT_UNIQUE_STRING]
The following values should be passed as GET parameters:
client_id
- issued when you created your app (required)scope
- permissions to request (see Scopes) (required)redirect_uri
- URL to redirect back to (see step 4 of Create you App) (optional)state
- unique string to be passed back upon completion (optional)
If the redirect_uri
of your application is configured in the settings, the redirect_uri
parameter may be omitted. If present, it should match the one statically defined.
If the state
parameter is set in the request it will be returned to the application as part of the redirect_uri
. Your app can use it for making sure the response belongs to a request initiated by the same user.
2. Authorization Grant - Redirection to your server with an authorization code
2.1 The user is prompted to log in and to authorize your application
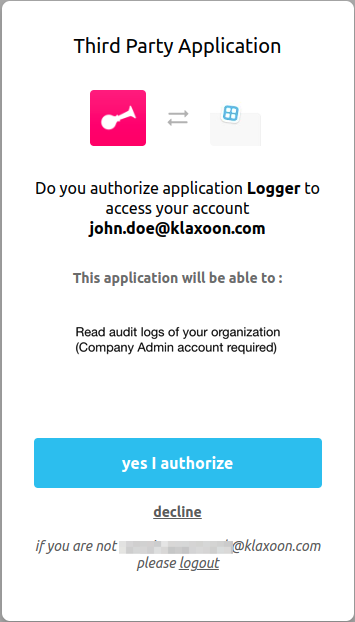
Klaxoon OAuth2 dialog
2.2 If the user authorizes your app, Klaxoon will redirect back to your specified redirect_uri
with a temporary authorization code in a GET parameter.
3. Authorization Grant - Exchange the authorization code for an access token
Exchange the authorization code for an access token and refresh token from the https://api.klaxoon.com/auth/oauth2/token?
API method
client_id
- issued when you created your app (required)client_secret
- issued when you created your app (required)code
- a temporary authorization code (required)redirect_uri
- must match the originally submitted URI (required)grant_type
- must beauthorization_code
(required)
Request example
curl --request POST \
--url https://api.klaxoon.com/auth/oauth2/token \
--header 'accept: application/json' \
--header 'Content-Type: application/json' \
--data '
{
"client_id": "[YOUR_CLIENT_ID]",
"client_secret": "[YOUR_CLIENT_SECRET]",
"code": "[YOUR_TEMPORARY_AUTHORIZATION_CODE]",
"redirect_uri": "[YOUR_REDIRECT_URI]",
"grant_type": "authorization_code"
}'
Response example
{
"expires_in": 7200,
"access_token": "n2yMa7Q...IUoP",
"token_type": "bearer",
"refresh_token": "mFfe...P8jX"
}
4. Use the access token in request to Klaxoon API
The access token allows you to make requests to the Klaxoon API.
Klaxoon uses Bearer authentication which is an HTTP authentication scheme that involves security tokens called bearer tokens. “Bearer authentication” can be understood as “give access to the bearer of this token.”
When making a request to Klaxoon APIs you need to include the following header in your API calls:
Header Parameter | Value |
---|---|
Authorization | Bearer [YOUR_ACCESS_TOKEN] |
Request example :
curl --request GET \
--url 'https://api.klaxoon.com/v1/auditlogs?page=1&perPage=50' \
--header "Authorization: Bearer n2yMa7Q...IUoP"
Response example :
{
"items": [
{
"id": "0651b6d9-0a15-4658-b372-dee5ab67ec71",
"actionDate": "2022-06-20T20:20:20.123Z",
"action": "ORGANIZATION_INVITATION_ACCESS_RESTRICTED",
"author": {
"id": "8e04eacd-cf33-42b8-85d7-7340f9a2bca8",
"type": "USER",
"email": "johndoe@company.com",
"companyId": "0913eaf2-c834-4d62-8794-c5f83a13e45e",
"companyName": "The author company",
"ipAddress": "0.0.0.1",
"requestId": "ea7670be-c3bd-4a00-8dc2-a249719ebc7c",
"userAgent": "Mozilla/5.0 (X11; Linux x86_64; rv:101.0) Gecko/20100101 Firefox/101.0",
"isExternal": true
},
"affected": {
"id": "965f7af5-5351-4042-a56a-1964fe2946b0",
"type": "BOARD",
"email": "jackdoe@company.com",
"companyId": "3c05e7d9-847f-4438-8d46-3f9168554d95",
"companyName": "The affected company",
"isExternal": true
},
"content": {
"message": "<Reason>",
"url": "https://klaxoon.com"
}
}
],
"self": "https://api.klaxoon.com/audit/v1/logs?page=3",
"first": "https://api.klaxoon.com/audit/v1/logs",
"prev": "https://api.klaxoon.com/audit/v1/logs?page=2",
"next": "https://api.klaxoon.com/audit/v1/logs?page=4"
}
5. Refresh Token
For security purposes when you request a token you retrieve as well the validity timelapse and a refresh token.
Once your token has expired, your applications must use the refresh token to "refresh" the expired access token and get a new one.
Refresh an access token from the https://api.klaxoon.com/auth/oauth2/token
API method:
grant_type
- refresh_token (required)refresh_token
- the refresh token retrieved while requesting the initialaccess_token
(required)client_id
- issued when you created your app (required)client_secret
- issued when you created your app (required)
Request example :
curl --request POST \
--url https://api.klaxoon.com/auth/oauth2/token \
--header 'accept: application/json' \
--header 'Content-Type: application/json' \
--data '
{
"grant_type": "refresh_token",
"refresh_token": "[YOUR_REFRESH_TOKEN]",
"client_id": "[YOUR_APP_ID]",
"client_secret": "[YOUR_CLIENT_SECRET]",
}'
Response example :
{
"expires_in": 7200,
"access_token": "Csi...MEjr",
"token_type": "bearer",
"refresh_token": "OkF...x2T"
}
Access tokens expire after 7200 seconds. Refresh tokens expire after 14 days.
Updated 11 months ago